Verilog, standardized as IEEE 1364, is a hardware description language (HDL) used to model electronic systems. It is most commonly used in the design and verification of digital circuits at the register-transfer level of abstraction. It is also used in the verification of analog circuits and mixed-signal circuits
Syntax of verilog:-
module module_name(variable1,variable2......);
input variable1;
---------------
---------------
output variableN;
always @(variables)
begin
----------------------
instructions;
----------------------
end
endmodule
-
Lets see one simple well known example
Example: AND gate
symbol and truth-table
>
Syntax of verilog:-
module module_name(variable1,variable2......);
input variable1;
---------------
---------------
output variableN;
always @(variables)
begin
----------------------
instructions;
----------------------
end
endmodule
-
Lets see one simple well known example
Example: AND gate
symbol and truth-table
>
// AND gate design
module andgate(a,
b,
y); // module declaration
input a; // initialising input/output variables
input b;
output y;
reg y;
always@(a,b) // always monitor a and b
begin
if(a==1'b1 & b==1'b1) // checking the condition a=b=1
begin
y = 1'b1; // if yes then y=1
end else
y = 1'b0; // if not y=0
end
endmodule
OUTPUT:
-
Example: OR Gate
symbol and truth table
// OR Gate
module OR_gate(a,
b,
y);
input a;
input b;
output y;
reg y;
always @(a,b)
begin
if (a==1'b0 & b==1'b0)
begin
y = 1'b0;
end else
y = 1'b1;
end
endmodule
OUTPUT:
-
Example: Inverter using Switch case
symbol and table
// CMOS Inverter using switch case
module cmos_inv(inv_in,
inv_out
);
input inv_in;
output inv_out;
reg inv_out;
always @(inv_in)
begin
case(inv_in)
1'b0 : inv_out = 1'b1;
1'b1 : inv_out = 1'b0;
endcase
end
endmodule
OUTPUT:
-
Example: Full Adder
symbol and functional table
// full adder gate-level design
module full_adder_gate(A,
B,
C,
s_out,
c_out);
input A;
input B;
input C;
output s_out;
output c_out;
reg s_out;
reg c_out;
always @(A,B,C)
begin
s_out = A^B^C;
c_out = (A & B) | (B & C) | (C & A);
end
endmodule
// full_adder using data_flow model (behavioral model)
module full_adder_dataflow(data_ins,
s_out,
c_out
);
input [2:0] data_ins;
output s_out;
output c_out;
reg s_out;
reg c_out;
always @(data_ins)
begin
if(data_ins == 3'b000)
begin
s_out = 1'b0;
c_out = 1'b0;
end if(data_ins == 3'b001)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b010)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b011)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b100)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b101)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b110)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b111)
begin
s_out = 1'b1;
c_out = 1'b1;
end
end
endmodule
OUTPUT:
-
Example: 2:4 Decoder
// 2x4 Decoder using if statement
module decoder_24(dec_ins,
dec_outs,
enable
);
input [1:0] dec_ins;
input enable;
output [3:0] dec_outs;
reg [3:0] dec_outs;
always @(enable or dec_ins)
begin
if (enable==1'b1) begin
if (dec_ins == 2'b00) begin
dec_outs = 4'b0001;
end if (dec_ins == 2'b01) begin
dec_outs = 4'b0010;
end if (dec_ins == 2'b10) begin
dec_outs = 4'b0100;
end if (dec_ins == 2'b11) begin
dec_outs = 4'b1000;
end
end
end
endmodule
// 2x4 decoder using case statement
module decoder_case(dec_ins,
dec_outs,
enable);
input [1:0] dec_ins;
input enable;
output [3:0] dec_outs;
reg [3:0] dec_outs;
always @ (enable or dec_ins)
begin
dec_outs = 4'b0000;
if (enable)
case(dec_ins)
2'b00 :
dec_outs = 4'b0001;
2'b01 :
dec_outs = 4'b0010;
2'b10 :
dec_outs = 4'b0100;
2'b11 :
dec_outs = 4'b1000;
endcase
end
endmodule
OUTPUT:
-
Example: 4:2 Encoder
// 4x2 encoder using case
module encoder_case(enc_ins,
enable,
enc_out
);
input [3:0] enc_ins;
input enable;
output [1:0] enc_out;
reg [1:0] enc_out;
always @(enable,enc_ins)
begin
if (enable) begin
case(enc_ins)
4'b0001 : enc_out = 2'b00;
4'b0010 : enc_out = 2'b01;
4'b0100 : enc_out = 2'b10;
4'b1000 : enc_out = 2'b11;
default : enc_out = 2'b00;
endcase
end
end
endmodule
// 4x2 Encoder using if statement
module encoder_if(enc_in,
enc_out,
enable
);
input [3:0] enc_in;
input enable;
output [1:0] enc_out;
reg [1:0] enc_out;
always @(enable, enc_in)
begin
if (enable) begin
if (enc_in == 4'b0001) begin
enc_out = 2'b00;
end else if (enc_in == 4'b0010) begin
enc_out = 2'b01;
end else if (enc_in == 4'b0100) begin
enc_out = 2'b10;
end else if (enc_in == 4'b1000) begin
enc_out = 2'b11;
end else
enc_out = 2'b00;
end
end
endmodule
OUTPUT:
-
Example: 4x1 Multiplexer
// 4x1 mux using case
module mux_case(I0,
I1,
I2,
I3,
S,
y);
input I0;
input I1;
input I2;
input I3;
input [1:0] S;
output y;
reg y;
always @(S,I0,I1,I2,I3)
begin
case(S)
2'b00 : y = I0;
2'b01 : y = I1;
2'b10 : y = I2;
2'b11 : y = I3;
endcase
end
endmodule
OUTPUT:
-
module andgate(a,
b,
y); // module declaration
input a; // initialising input/output variables
input b;
output y;
reg y;
always@(a,b) // always monitor a and b
begin
if(a==1'b1 & b==1'b1) // checking the condition a=b=1
begin
y = 1'b1; // if yes then y=1
end else
y = 1'b0; // if not y=0
end
endmodule
OUTPUT:
-
Example: OR Gate
symbol and truth table
// OR Gate
module OR_gate(a,
b,
y);
input a;
input b;
output y;
reg y;
always @(a,b)
begin
if (a==1'b0 & b==1'b0)
begin
y = 1'b0;
end else
y = 1'b1;
end
endmodule
OUTPUT:
-
Example: Inverter using Switch case
symbol and table
// CMOS Inverter using switch case
module cmos_inv(inv_in,
inv_out
);
input inv_in;
output inv_out;
reg inv_out;
always @(inv_in)
begin
case(inv_in)
1'b0 : inv_out = 1'b1;
1'b1 : inv_out = 1'b0;
endcase
end
endmodule
OUTPUT:
-
Example: Full Adder
symbol and functional table
// full adder gate-level design
module full_adder_gate(A,
B,
C,
s_out,
c_out);
input A;
input B;
input C;
output s_out;
output c_out;
reg s_out;
reg c_out;
always @(A,B,C)
begin
s_out = A^B^C;
c_out = (A & B) | (B & C) | (C & A);
end
endmodule
// full_adder using data_flow model (behavioral model)
module full_adder_dataflow(data_ins,
s_out,
c_out
);
input [2:0] data_ins;
output s_out;
output c_out;
reg s_out;
reg c_out;
always @(data_ins)
begin
if(data_ins == 3'b000)
begin
s_out = 1'b0;
c_out = 1'b0;
end if(data_ins == 3'b001)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b010)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b011)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b100)
begin
s_out = 1'b1;
c_out = 1'b0;
end if(data_ins == 3'b101)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b110)
begin
s_out = 1'b0;
c_out = 1'b1;
end if(data_ins == 3'b111)
begin
s_out = 1'b1;
c_out = 1'b1;
end
end
endmodule
OUTPUT:
Example: 2:4 Decoder
// 2x4 Decoder using if statement
module decoder_24(dec_ins,
dec_outs,
enable
);
input [1:0] dec_ins;
input enable;
output [3:0] dec_outs;
reg [3:0] dec_outs;
always @(enable or dec_ins)
begin
if (enable==1'b1) begin
if (dec_ins == 2'b00) begin
dec_outs = 4'b0001;
end if (dec_ins == 2'b01) begin
dec_outs = 4'b0010;
end if (dec_ins == 2'b10) begin
dec_outs = 4'b0100;
end if (dec_ins == 2'b11) begin
dec_outs = 4'b1000;
end
end
end
endmodule
// 2x4 decoder using case statement
module decoder_case(dec_ins,
dec_outs,
enable);
input [1:0] dec_ins;
input enable;
output [3:0] dec_outs;
reg [3:0] dec_outs;
always @ (enable or dec_ins)
begin
dec_outs = 4'b0000;
if (enable)
case(dec_ins)
2'b00 :
dec_outs = 4'b0001;
2'b01 :
dec_outs = 4'b0010;
2'b10 :
dec_outs = 4'b0100;
2'b11 :
dec_outs = 4'b1000;
endcase
end
endmodule
OUTPUT:
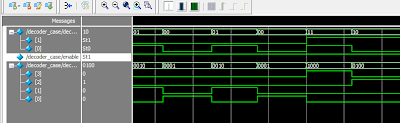
-
Example: 4:2 Encoder
// 4x2 encoder using case
module encoder_case(enc_ins,
enable,
enc_out
);
input [3:0] enc_ins;
input enable;
output [1:0] enc_out;
reg [1:0] enc_out;
always @(enable,enc_ins)
begin
if (enable) begin
case(enc_ins)
4'b0001 : enc_out = 2'b00;
4'b0010 : enc_out = 2'b01;
4'b0100 : enc_out = 2'b10;
4'b1000 : enc_out = 2'b11;
default : enc_out = 2'b00;
endcase
end
end
endmodule
// 4x2 Encoder using if statement
module encoder_if(enc_in,
enc_out,
enable
);
input [3:0] enc_in;
input enable;
output [1:0] enc_out;
reg [1:0] enc_out;
always @(enable, enc_in)
begin
if (enable) begin
if (enc_in == 4'b0001) begin
enc_out = 2'b00;
end else if (enc_in == 4'b0010) begin
enc_out = 2'b01;
end else if (enc_in == 4'b0100) begin
enc_out = 2'b10;
end else if (enc_in == 4'b1000) begin
enc_out = 2'b11;
end else
enc_out = 2'b00;
end
end
endmodule
OUTPUT:
-
Example: 4x1 Multiplexer
// 4x1 mux using case
module mux_case(I0,
I1,
I2,
I3,
S,
y);
input I0;
input I1;
input I2;
input I3;
input [1:0] S;
output y;
reg y;
always @(S,I0,I1,I2,I3)
begin
case(S)
2'b00 : y = I0;
2'b01 : y = I1;
2'b10 : y = I2;
2'b11 : y = I3;
endcase
end
endmodule
OUTPUT:
-
Read more...
No comments:
Post a Comment
comment here